System Information
-
Strapi Version: 3.6.2
-
Operating System: Linux
-
Database: PostgreSQL
-
Node Version: >=10.16.0 <=14.x.x
-
NPM Version: >=6.0.0
-
Yarn Version: 1.22.10
I’m working on a blog page for my website that’s based on Next.js, written on TypeScript and that uses Strapi as CMS API.
The following code snippet from my front-end is giving me a Server Error that says TypeError: posts.map is not a function
which I have no idea why.
type BlogPageProps = {
posts: PostData[];
};
const BlogPage = ({ posts }: BlogPageProps) => {
useEffect(() => {
AOS.init({ duration: 700 });
}, []);
return (
<>
<SEO title={`Blog | ${SITE_NAME}`} />
<AnimationContainer animation="appearFromAbove">
{posts.map((post) => (
<PostCard effect="fade-up" key={post.id} post={post} />
))}
</AnimationContainer>
</>
);
};
export default BlogPage;
The PostData[] is only a type that consists of the following parameters :
export type PostData = {
id: PostID;
title: string;
content: string;
slug: string;
author: PostAuthor;
category: PostCategory;
created_by: PostCreatedBy;
updated_by: PostCreatedBy;
created_at: string;
updated_at: string;
cover: PostCover;
};
I’m thinking this must has something to do with the API itself, not the code from the front-end development because when I change the API_URL (currently set to https://major-harpia.herokuapp.com/) to one that a friend uses as his API, it runs fine.
More specifically, I’m suspecting on the following causes :
- PostgreSQL - I’m not sure if I configured it properly on heroku because all I did was install a add-on
- JWT Token - Since I’ve cloned the repository from someone else, I have not generated any JWT token of my own to use in the API.
Please, could someone clarify this issue? It’s driving me nuts.
Can you provide more information as to what is trying to be done here? The PostData, is that just a GET request on REST or a POST request (to fetch data) on GraphQL?
Not sure what you mean @DMehaffy . If you’d like I can share the website and API repository link to give you some more context :
API : https://github.com/gabrielpulga/site-pessoal-strapi-api
Website : https://github.com/gabrielpulga/site-pessoal
Perhaps this can be useful information :
When I run “yarn develop” or “npm start” on the API project, I get an error :
debug ⛔️ Server wasn't able to start properly.
error Error: connect ECONNREFUSED 127.0.0.1:5432
at TCPConnectWrap.afterConnect [as oncomplete] (net.js:1146:16)
That means the Strapi server can’t connect to the PostgreSQL server
@DMehaffy Ok, I’ve managed to install PostgreSQL and run the API from my machine. Nothing changed as there are no new files added to the project from this.
The blog page loads properly when I use https://protected-eyrie-31544.herokuapp.com as API_URL but doesn’t load when I use https://major-harpia.herokuapp.com which is my own API, pretty much identical to the protected-eyrie one.
Why is this happening? Could it have something to do with the jwt token used in server.js?
module.exports = ({ env }) => ({
host: env('HOST', '0.0.0.0'),
port: env.int('PORT', 1337),
admin: {
auth: {
secret: env('ADMIN_JWT_SECRET', '7cb260a16ee334dcecc1e64c07d72348'),
},
},
});
I haven’t generated the token above so I don’t know what exactly it does or if it’s working properly.
So, I decided instead of running the API from https://major-harpia.herokuapp.com, I’ll run it from my localhost to see if I could troubleshoot the errors better.
This looks like progress. I think the response body is returning HTML, not JSON.
EDIT : Okay, I’m dumb and it turned out it was the wrong localhost address that I was using (:3000 instead of :1337).
When I use :1337, I get the same error as before, specified in the topic :
I’m not sure why the .map() isn’t working since that’s not part of the Strapi code-base (guessing your frontend) but are you able to start the Strapi server and are you getting data (say if you test with postman)
@DMehaffy Yes, I’m starting the Strapi server with zero errors and it seems like every call I make to the API receives a GET request but a 400 response error.
Those logs tell the story, something is injecting an extra /
into the request path
and your request structure is wrong, specifically the posts?&_sort
There should be no &
after the ?
You’re right @DMehaffy. There was a / at the end of the API_URL that was messing up the link and ALSO I needed to set the permissions “find” and “findOne” to display the posts through public access.
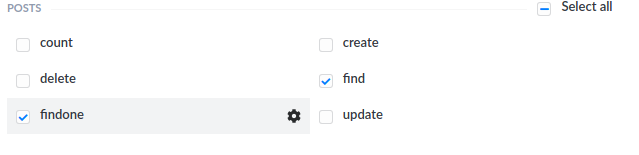
1 Like